Installation & Setup
Welcome to the React SDK setup guide! If you’re looking for guides for other frameworks, check out the Next.js SDK Setup, or the JavaScript SDK Setup.
Before getting started, make sure you have a React project setup. We show an example here of a Vite React project with React Router.
Create API keys
If you haven’t already, register a new account on Stack, create a project in the dashboard, create a new API key from the left sidebar, and copy the project ID, publishable client key, and secret server key.
Create stack.ts
file
Create a new file stack.ts
in your root directory and fill it with the following Stack app initialization code:
Update App.tsx
Update your App.tsx
file to wrap the entire app with a StackProvider
and StackTheme
and add a StackHandler
component to handle the authentication flow.
Done!
That’s it! Stack is now configured in your React project. If you start your React app and navigate to http://localhost:5173/handler/sign-up, you will see the sign-up page.
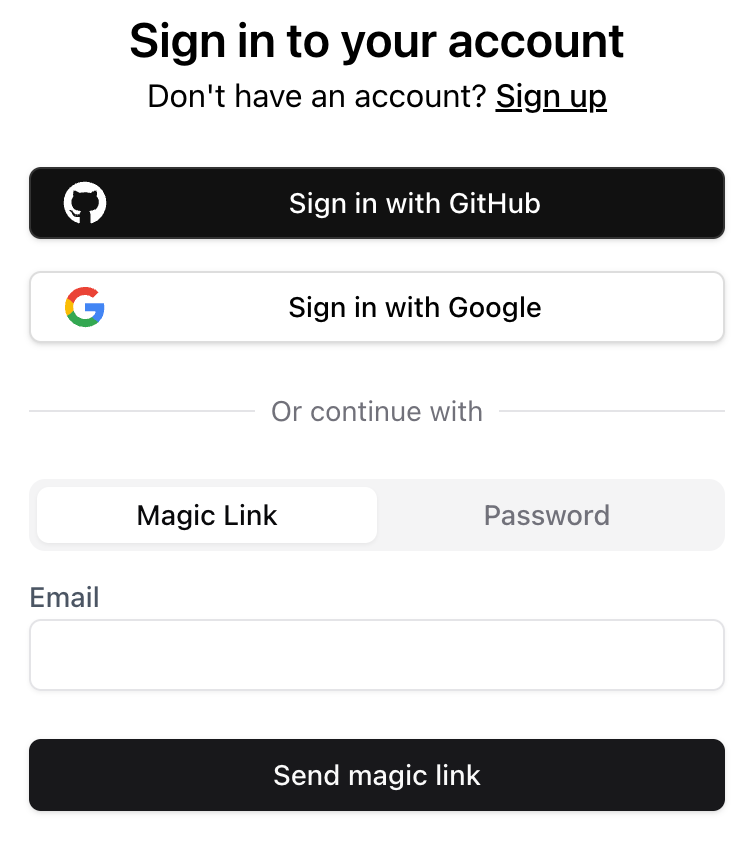
After signing up/in, you will be redirected back to the home page. We will show you how to add user information to it in the next section. You can also check out the http://localhost:5173/handler/account-settings page which looks like this: